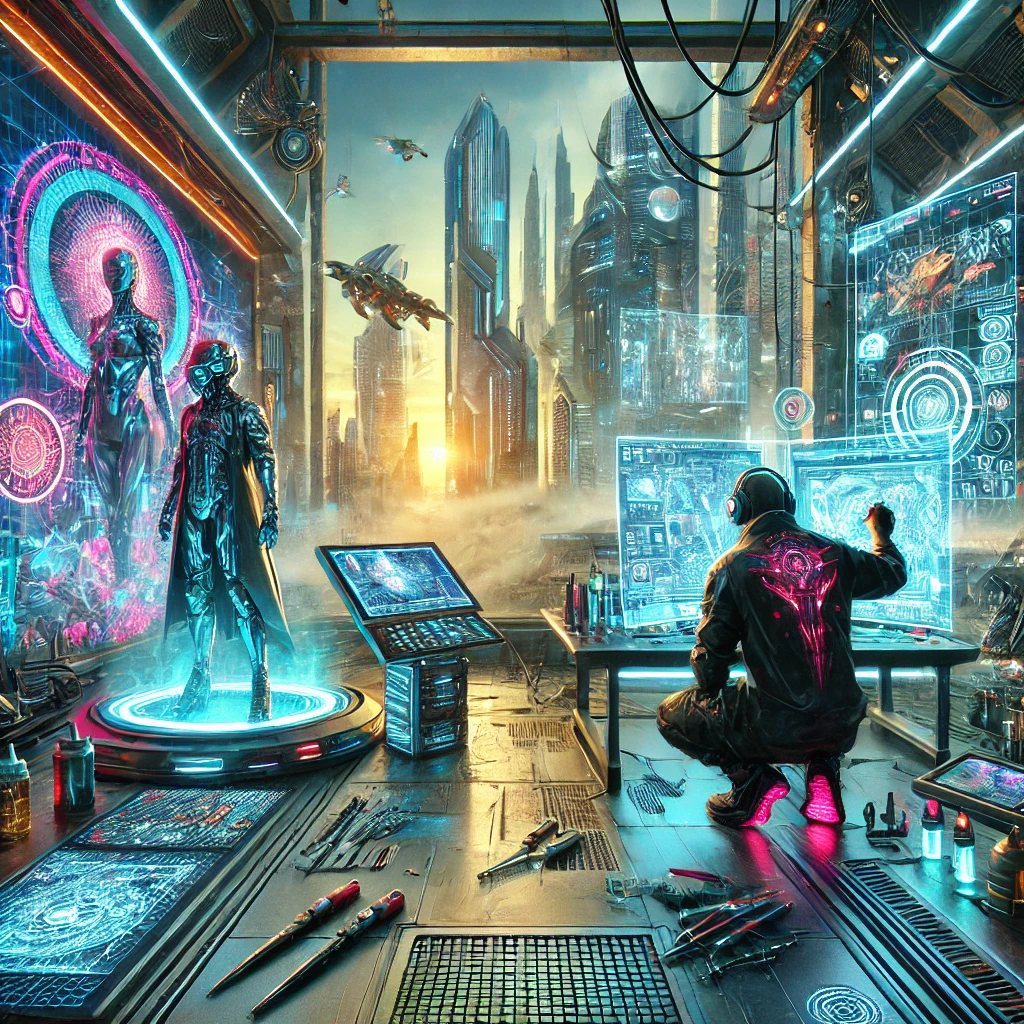
You can customize the way Rozz is triggered in your HTML page by following these instructions. You might want to do this if you wish to use another button than our default ‘Ask Anything’ button.
HTML Structure
Your HTML structure should include the rozz-searchbox
component and a clickable element that will trigger the searchbox. The initial-status attribute should be set to “link” (see https://rozz.site/installing-rozz-on-your-website/ for details on configuration):
<rozz-searchbox id="rozz-searchbox" initial-status="link"></rozz-searchbox>
Here’s what the rest of the code can look like:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Rozz Searchbox Example</title> <script src="https://rozzum-bucket.rozz.site/rozz-searchbox.js"></script> </head> <body> <div id="rozz-container"> <rozz-searchbox id="rozz-searchbox" initial-status="link"></rozz-searchbox> </div> <div> <img src="https://your-bucket.amazonaws.com/example.com.png" id="example.com" data-domain="example.com" alt="Search my website" title="Example Website" style="cursor: pointer;"> </div> <script> document.getElementById('example.com').addEventListener('click', function() { const domain = this.dataset.domain; const searchbox = document.getElementById('rozz-searchbox'); if (searchbox) { searchbox.openClickLink(domain); // Opens and connects the searchbox } }); </script> </body> </html>
Explanation
HTML Structure
- The
<div id="rozz-container">
contains therozz-searchbox
element.
- The image element (
<img>
) represents the clickable source that will trigger the searchbox. It has anid
, adata-domain
attribute, and other standard attributes.
Javascript
- An event listener is added to the image element with the
id
ofexample.com
. - When the image is clicked, the
data-domain
attribute of the clicked image is retrieved. - The
openClickLink(domain)
method of therozz-searchbox
element is called with the retrieved domain.
Customizing the Domain
To customize the domain, you can change the data-domain
attribute of the image element. The JavaScript will automatically use the updated domain value when the image is clicked.
<img src="https://example.com/logo.png" id="example" data-domain="example.com" alt="Example Domain" title="Example Domain" style="cursor: pointer;">
Update the JavaScript to reference the new id
:
document.getElementById('example').addEventListener('click', function() { const domain = this.dataset.domain; const searchbox = document.getElementById('rozz-searchbox'); if (searchbox) { searchbox.openClickLink(domain); } });
That’s how you can integrate and use the rozz-searchbox
component in your HTML page with a single clickable element that sets the data-domain
attribute dynamically based on user interaction. I hope this helps!